I'm struggling with adding the ability for the user to purchase new producers.
my task: If user presses the key that corresponds to the id of a producer and they have enough gold to by it, then
we should:
1) Deduct the cost of the producer from the gold balance
2) Increase the cost of the next producer by multiplying the current cost by the growthRate
3) Increment the count of the producer that was purchased
my handlers.js looks like:
import { updateGold } from "./functions.js"
import { config } from './constants.js'
export const handleKeyPress = (term, state) => {
return (name, matches, data) => {
let key = String.fromCharCode(data.code)
if (key === 'g' || key === 'G') {
state.gold+=1 }
updateGold(term, state)
for (let i=0; i<config.producers.length; i++) {
if (key === config.producers.id && config.gold > config.producers.cost) {
config.gold -= config.producers.cost
config.producers[i].cost * config.producers[i].growthRate
config.producers[i].count++
}
}
}
}
errors: 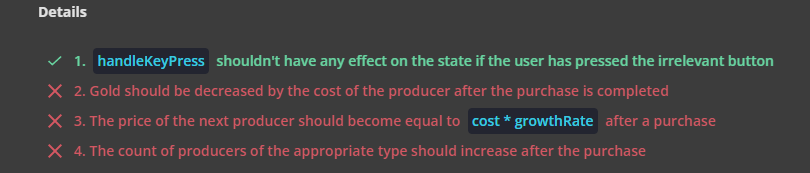